Quick Overview of JavaScript Array Methods
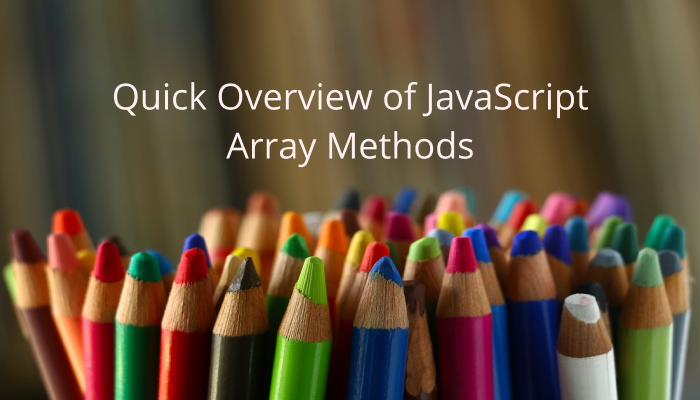
Quick Overview of JavaScript Array Methods
To make JavaScript array manipulation easier, we should use array methods to make our work easier and the code cleaner.
We’ll look at some most commonly used array methods and how we can use them.
Looping Through Each Entry with Array.prototype.forEach
We can loop through all array entries with the .forEach method.
It takes one argument, which is a callback function. The callback function takes up to 4 arguments.
The first argument is the currentValue which is being iterated through.
The second argument is the index of the currentValue in the array that forEach is called on. This is an optional argument.
The third argument is the array
that it’s being called on, which is again optional.
Finally, the fourth argument is the this
value to use when calling the callback function. We usually don’t need to worry about this.
It returns nothing.
For example, we can use it as follows:
1 2 3 4 | const arr = [1, 2, 3]; arr.forEach((num, index) => { console.log(`Current entry: ${num}. Current index: ${index}`); }) |
In the code above, we call forEach on arr with a callback passed in to log the entry that’s currently being accessed and the index of that entry.
Filtering Array Items with Array.prototype.filter
We can create an array that has the subset of the items of the original array by including the items that meet the condition we want by using the filter
method.
It takes a callback function as an argument. The callback function can have the following parameter:
- element – the current element being processed in the array
- index – an optional parameter that has the index of the current array element being processed.
- array – an optional parameter that the filter method was called on.
- thisArg – an optional parameter that lets us sets the value of this inside the callback.
Our callback should return the condition that needs to be met for the items to be included with the returned array.
It returns a new array with the entries that meet the condition that we returned in the callback.
For example, given that we have an array of to-do items and we want to get the ones that are done. we can use filter
to do that as follows:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 | const todos = [{ name: 'eat', done: true }, { name: 'drink', done: true }, { name: 'sleep', done: false }, ]; const doneTodos = todos.filter((todo) => todo.done) |
In the code above, we have the todos
array.
Then, we call filter on it by writing:
1 | const doneTodos = todos.filter((todo) => todo.done); |
which gets the items that have the done
property set to true
, put them in a new array, and then return it.
If we run console.log on doneTodos, we get:
1 2 3 4 5 6 7 8 9 10 | [ { "name": "eat", "done": true }, { "name": "drink", "done": true } ] |
Mapping Array Items with the Array.prototype.map Method
We can call the map
method on an array to map each entry of an array from one thing to another.
It returns a new array with the mapped entries.
It takes a callback function which takes the following parameters:
- currentValue – the current element being processed in the array
- index – the index of the current element being processed in the array. It’s an optional parameter.
- array – the array which map
is being called on. It’s also optional
- thisArg – the thisvalue which we want to set inside the callback, which is also optional.
The callback is called on all elements once, including undefined . Although it’s not called for missing elements in the array. That is, indexes that have never been set, array entries that have been deleted, or have never been assigned a value will not be called.
Since it returns a new array, it shouldn’t be called if we want to manipulate an existing array’s entry in place.
If we want to modify values in place, we have to use loops like the for
or for-of
loops.
For example, we can use it to map a number array by multiplying each entry by 2 as follows:
1 2 | const nums = [1, 2, 3]; const doubledNums = nums.map(num => num * 2); |
Then we get [2, 4, 6] as the value of doubledNums .
We can pass in any function that takes a value and then returns it in the way that we want.
For example, JavaScript has a built-in Numberfunction that takes a string and then returns a number that the string includes.
Since the first parameter is the thing that we want to iterate through and it returns something we want, we can pass in the Number
function as the callback of map .
For instance, we can write:
1 2 | const stringNums = ['1', '2', '3']; const nums = stringNums.map(Number); |
Combining Array Elements into One Value with Array.prototype.reduce
We can use the reduce method to combine values in an array into one value.
The syntax of reduce is as follows:
1 | arr.reduce(callback( accumulator, currentValue[, index[, array]] )[, initialValue]) |
The reduce method takes a reducer function that takes up to 4 arguments:
- accumulator – the accumulator value, or the value of the values that are combined so far
- currentValue – the current value being processed and will be incorporated into the
acc
value - index – the current index of the item being processed
- array – the array that the reduce method is being called on
In addition to the callback function, it also takes a second argument so that we can set an initialValue
for the accumulator
.
If no initialValue
is specified then the first element of the array will be used and skipped.
Calling reduce
with on an empty array with no initialValue
will cause a TypeError
will be thrown.
For example, we can use it to add an array of numbers together as follows:
1 2 | const nums = [1, 2, 3, 4, 5, 6] const sum = nums.reduce((a, b) => a + b, 0); |
Then we get 21 as the value of sum
since added all the values together with reduce and set the initialValue to 0.
Conclusion
We can use the forEach method to loop through each entry.
If we want a filtered array of items according to the condition we specify, we can use the filter method.
To map each entry of an array from one thing to another, we can use the map method.
Finally, if we want to combine all array entries into one value, we can use the reduce method.
No comments yet