How I Built an Admin Dashboard with Python Flask
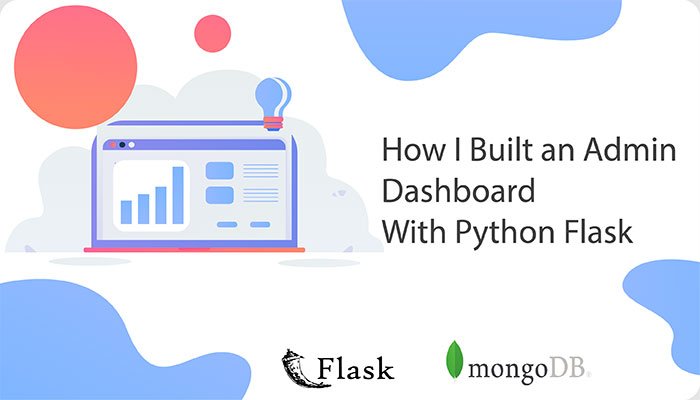
How I Built an Admin Dashboard with Python Flask
Before I jump into the the full details and guidelines of how I built this Admin Dashboard, let’s know what Python Flask is and why I decided to build this Admin Dashboard with Flask.
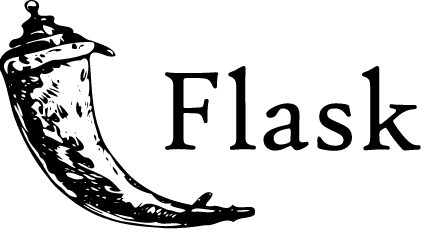
Flask
Flask is a lightweight Python web framework that allows users to build web application faster and easier and also give its users as much flexibility as possible in building and scaling their web applications.
Flask is a micro-framework, hence, it allows its users to use their preferred extensions and packages in building their web applications. Unlike other frameworks, Flask doesn’t force its user to follow a particular layout hence its flexibility.
It’s also important to note that Flask has a large community that provide answers to different questions and issues you might face as a Flask developer, they also build and provide so many open source packages for smooth sailing while building a flask web application. Flask has an in-built web server that allows the Python application to process and also makes the Python application accessible to the public.
Now that we have a clear overview of what Flask is, I’ll talk about MongoDB, the database used in storing the user data gotten on registration on this Admin Dashboard.
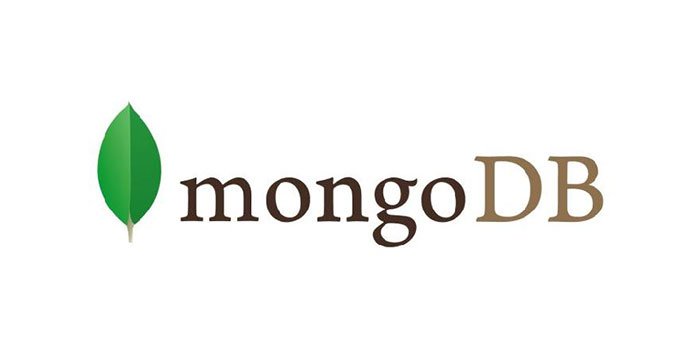
MongoDb
MongoDB is an open source document-oriented NoSQL database management system used for storing data in flexible, JSON-like documents. Unlike some other SQL databases, MongoDB doesn’t use relational table-based structure.
MongoDB is a very strong and powerful and flexible system that uses dynamic schemas in JSON-like documents called BSON, this makes it very fast and easy for data integration in different types of application. MongoDB is scalable and has high performance and availability for even large and complex infrastructures.
Features of the Application
The features of the Admin Dashboards include:
- MongoDB database
- Session-based authentication (Login and Registration)
- SMTP Integration on signup and login
- Gunicorn Deployment script (For Heroku)
- Free SB-Admin 2 Dashboard Theme
- Illustrations from Undraw.co
Without further ado, let’s get into how this web application was built.
Installations
There are certain requirements needed to be able to create or run a Flask application. Some of these requirements are:
Once you have these requirements installed on your laptop, you can go ahead to creating your Flask application.
Setting up the Flask Application
The first step to creating a Flask application would be to create a directory/folder where the files and folders of the application will be. To create a working directory go to your terminal and run:
1 2 | mkdir flask-admin cd flask-admin |
Running the line above creates the working directory and help you navigate into the directory.
The next step is to create a virtual environment where our dependencies will be installed including our Flask package. To create and use a virtual environment, run the following lines in your terminal while in the working directory of your application:
– Install the python virtual environment with pip:
1 | pip install virtualenv |
– Create a virtual environment for our application:
1 | virtualenv venv |
– Activate the virtual environment:
1 | source venv/bin/activate |
Having created and activated a virtual environment, we can then clone the Github repository of this application to install the required packages and also dive into the different sections of the application to understand how I built it.
After cloning the application from GitHub, the next step is to install the packages in the requirements.txt file. Do that by running the following line in your terminal:
1 | pip install -r requirements.txt |
Setting up SMTP and MongoDB database servers
There are a lot of mail server that can be used to send emails programmatically from our Flask application. In this application, I made use of Sendgrid, but you can make use of any mail server you are comfortable with.
There is a package, Flask-Mail, that provides a plug and play implementation of SMTP in Python Flask. All you need to do is to install the package (it’s already included in the requirements.txt file) then provide the Mail Server, Mail port (it’s mostly usually either 465 or 587 depending on the security of the mail server), Mail username, Mail password(should never be stored in the application but rather as an environment variable with os.environ).
As said earlier, MongoDB is the database used for this application because of its ease of use and speed for data integration. Because we plan to host the application, I created a MongoDB Atlas account on the MongoDB website and created a new database for this application online rather than setting up MongoDB locally on my machine. But if you don’t plan to host this application to the general public, you can set up MongoDB locally and create a database for the application.
All dependencies are now installed and we’re almost ready to run our Flask Admin Dashboard :). Before we run our application, it’s important that we understand all the sections of the application and how these sections come together to make this application function well.
Sections of the Application
Here is the project structure of our Flask application:
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 | . ├── configuration │ └── __init__.py ├── helpers │ ├── database.py │ ├── hashpass.py │ └── mailer.py ├── model │ └── __init__.py ├── static │ ├── css │ ├── img │ ├── js │ ├── scss │ └── vendor ├── templates ├── views │ └── __init__.py ├── Procfile ├── app.py ├── requirements.txt │ |
Right about now, I’ll explain each folder and the contents of the folder.
- Configuration: It contains an __init__.py file where you input the mail smtp and database credentials. For security purpose, you mustn’t store plain passwords in this file.
- Helpers: This contains 3 files that have some functions that help to run the application smoothly and easily.
- database.py: This is where the MongoDB database connection is initiated and an instance of the database is created for use across different parts of the application.
- hashpass.py: This file houses the function for hashing passwords of user that register on the admin dashboard. We don’t want to store plain password in our database, so a function is created to hash passwords with help of a python package, hashlib.
- mailer.py: This is the package that holds the function that sends mails with certain parameters (subject, sender, recipient, body) provided.
- Model: The model is where we have the different querying functions for user authentication and other necessary authentications to make the application run effectively.
- Static: This is the file directory for all the static contents of the application theme. The css, images, javascript files and folders are kept in the static folder.
- Templates: This houses all the html files of the application theme.
- Views: The views is where we have the routes and html renderer that displays the web application to the end-user it also has endpoints for the Ajax program used in this application (for user authentication on the front-end).
- app.py: This is the main file of the application from where the application is run.
- Profile: The procfile is an text file that holds the command(s) for the process types in the application (it is important as it makes the application run on a server).
- requirements.txt: This file stores the packages and their version. It helps other developers that want to work on the application install the right version of the python packages needed to run the application.
Running the Application
If you followed the article up to this point, then I believe you have successfully set up the application, you understand the application and you are ready to run the application.
To run this flask application, navigate to your terminal with your virtual environment activated and run:
1 | python app.py |
You should see a url http:127.0.0.1:5000 provided to you in your terminal, open your preferred browser and navigate to that url or localhost:5000. You should see the login screen of the Admin Dashboard.
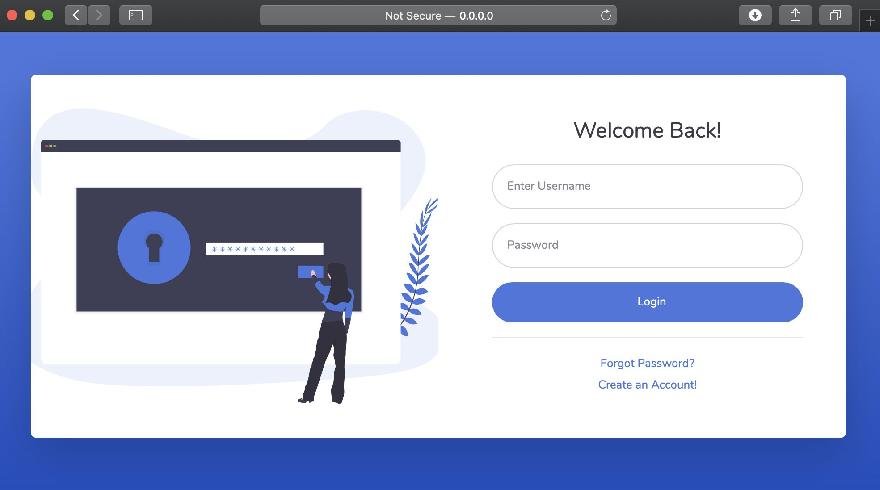
The Flask Admin Login Page
To be able to access the main page/dashboard, you need to create an account. After creating an account, a mail will be sent to your registered email that you have successfully created an account on Flask Admin Boilerplate.
This application has some javascript programs written with Jquery and Ajax to ensure that:
- Emails are unique and have not been used before
- Usernames are unique
- The password and confirm password match
- The username exists already before trying to login
Once you have been able to login with your right credentials, you will be able to see the main page of the dashboard. You should have something like this:
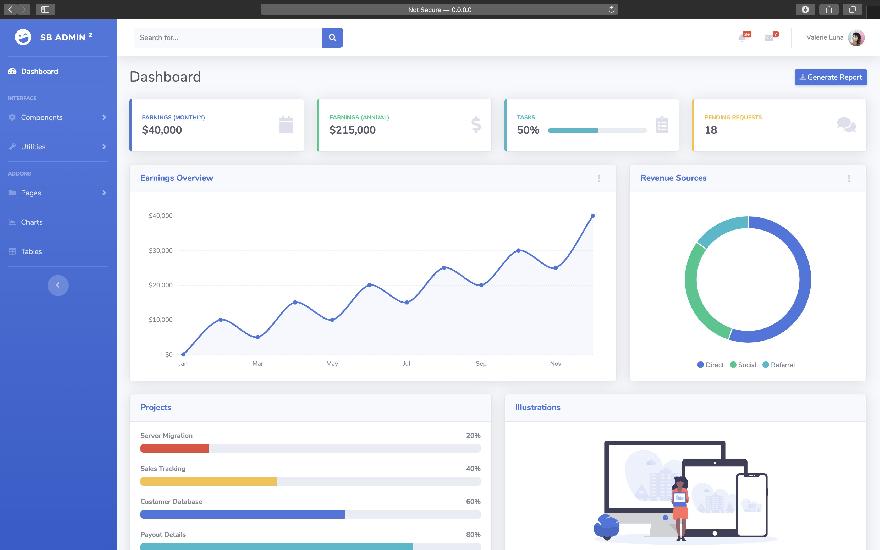
The Flask admin Dashboard page
Hosting on Heroku
To host this application on Heroku, go to Heroku website to create an account. Afterwards, create a new application.
For easy deployment of our application, create a GitHub repository and push the application to the repo. Go to the deploy tab on your Heroku application dashboard and connect the GitHub repository you created to the application. It will build the application for some minutes and once it’s done building you’ll be able to view your deployed application on https://your-app-name.herokuapp.com.
Finally, you can tweak and work your way around the web application. Fun fact: It’s absolutely open source and MIT Licensed.
Find the code to this repo here and you can also click here to view the hosted application on Heroku.
No comments yet