Top 18 Interview Questions for Python Developers
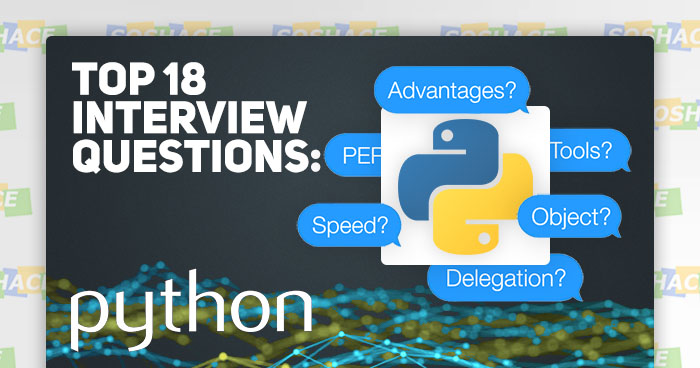
Asking the right questions to establish the right hiring strategy!
One of the most popular languages at the moment, Python is a great choice for a wide range of teams and products. Boasting features like incredible code readability, support for multiple programming paradigms and more, the language offers remarkable functionality and can be used in different spheres: web development, (data) science, desktop applications, and so on. Python developers, therefore, are in great numbers and ready to contribute to your project.
In this large pool of available job candidates, how to make sure that the person we hire matches the company’s standards? Whether it’s a remote Python developer or bringing them on-site, we need to conduct a technical interview in an effective manner, presenting our job candidate with interesting challenges. Our article will provide you with top 18 Python interview questions to make your hiring strategy better!
Python Theory and Fundamentals
A great Python developer is proficient in coding, but they can also operate with abstract ideas and concepts present in their programming language of choice. Knowing the strengths and weaknesses of Python, your job candidate can assess your project’s potential. In this section, we will test the Python developer’s core knowledge.
1. What advantages can Python offer?
Python’s advantages like readability and clean syntax are incredibly well-known, so we can discuss other great features of this language:
- Batteries included: boasting over 300 library modules, Python offers condensed (i.e. scripted) versions of many tasks that programmers usually do manually. Some of the modules included can: safely create temporary files, map files into memory, compress and decompress data, archive files, etc.
- Free + open-source + cross-platform: these three aspects allow for a Python’s massive adoption throughout the developer community and make sharing knowledge much easier
- Productivity: thanks to features like process integration, unit testing framework, and enhanced control capabilities, applications can improve their performance and productivity.
2. Python is considered to be a “slow” language. How can this criticism be addressed?
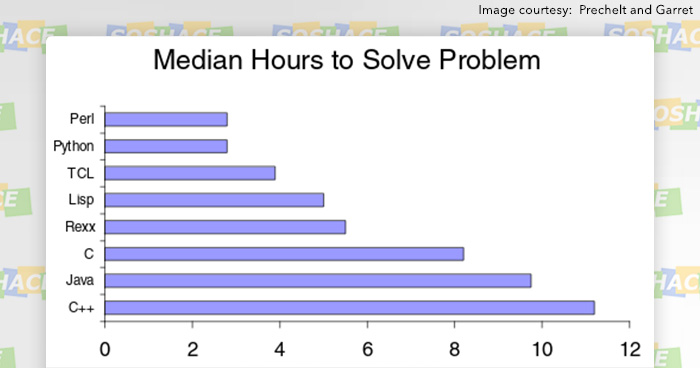
This is how many hours you’d need to write a string processing application
Python is indeed slower than low- and middle-level programming languages in terms of raw CPU performance — this is what we refer to when using the term “speed”. Oddly enough, CPU bottlenecks are, in fact, quite rare — this is what a study by Google shows. However, there is another aspect of this term: business speed in the form of time-to-market performance. Python’s advantages allow the developer to be more productive than their colleagues who use other languages. Python’s conciseness allows for writing less code which ultimately saves the developer’s time. Although “time spent writing code” is not the most objective metric, studies show that time spent per line of code is approximately the same in every programming language — which makes Python great productivity-wise. In other words, where Python lacks in the speed of execution, it compensates by the speed of development.
3. How can Python code be optimized?
Still, when it comes to performance and speed, the sky’s the limit — so we can utilize quite a few tricks to make our code run faster.
- When choosing between iterator-based tools and list-based tools, go for the former: the iterator forms are less memory-taxing and offer better scalability. Unless your project requires a real list, iterators are always the safe bet.
- Extension modules (
array, itertools,
andcollections.deque
) and built-in datatypes (lists, tuples, sets,
anddictionaries
) are coded via optimized C. We can design our application with this in mind, making it utilize these features to their fullest potential (an important caveat is: C-based modules offer less flexibility, so the developer should be informed about the functionality they may be forgoing) - Diagnostic tools: modules like hotshot and profile can be used to detect whether our application suffers from performance bottlenecks; the timeit module can compare the performance of different approaches to writing given lines of code
- Using threading, we can decrease the time it takes for our application to respond, effectively negating any wasted time waiting for I/O
- Certain external tools can also boost performance: e.g. Numpy is the go-to solution for high volume numeric work; syco and pyrex can help bring the code speed to the level of native code
- Using chained comparisons improves performance compared to using the “and” operator:
1 < 2 < 3
instead of1 < 2 and 2 < 3
4. Which tools can we use to make the code PEP 8-compliant? Why is it important?
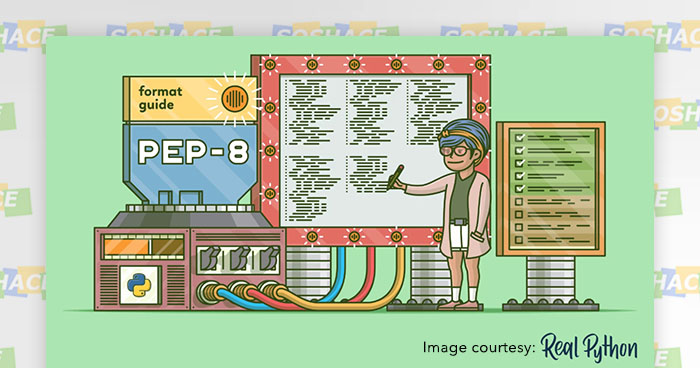
Writing Python code with style since 2001
Python Enhancement Proposal is a guideline for Python developers detailing how they should write “pythonic” code — one that conforms to Python standards of readability and cleanliness. It includes rules for naming conventions, code layout, indentation, commenting, using whitespaces, and more. Following PEP 8 guidelines is important because these rules are a standard that allows for writing better code faster. Especially in a collaborative environment, developers can work with PEP 8-compliant code created by their colleagues with ease: they know what to expect from each line of code, how to organize it properly, and so on.
To automate PEP 8 check-ups, we can use two types of software.
- Linters: pycodestyle, flake8. These programs analyze code and mark any found errors, providing feedback on how the errors can be fixed. Additionally, they can be utilized right in the code editor in the form of an editor extension, marking errors in real time as you type.
- Autoformatters: black, autopep8, yapf. These programs format the code to make it PEP 8-compliant automatically; for instance, they can set the line length limit.
5. How are arguments passed: by value or by reference?
In Python, everything is an object and all variables hold references to the objects. The reference values are linked to the functions; as a result you cannot change the value of the references. However, you can change the object if it is mutable.
6. What are the built-in types that Python provides? Which are mutable? Which are immutable?
Mutable built-in types:
- List
- Sets
- Dictionaries
Immutable built-in types:
- Strings
- Tuples
- Numbers
7. How can the “yield” keyword be utilized?
The yield keyword can turn any function into a generator, working like a standard return
keyword. However, it will always return a generator object. Also, a method can have multiple calls to the yield
keyword.
1 2 3 4 5 6 7 8 9 10 | def testgen(index): weekdays = ['sun','mon','tue','wed','thu','fri','sat'] yield weekdays[index] yield weekdays[index+1] day = testgen(0) print(next(day)) print(next(day)) #output: sun mon |
8. What is the optimal method of reading a large file (say, 8GB)?
First of all, we need to determine the file’s properties: what is the data’s nature? How do we operate it and what are we going to return? Is the file accessed in a sequential or random order? Then, we take certain precautions: operate on chunks of data instead of one byte at a time; keep the host machine’s memory in check.
1 2 3 | with open(...) as f: for line in f: # Do something with 'line' |
Using the with
statement, Python handles opening and closing the file. The file object f
is treated as an iterable by the for line in f
loop — this enables the automatic use of buffered I/O coupled with memory management (so that we do not have to worry about those issues).
9. What are the differences between multithreading and multiprocessing libraries in Python?
CPython (the standard default official interpreter) is not thread-safe when it comes to memory management. So, if we are running multiple threads, the GIL is a bottleneck — it only allows one thread to access memory at a time. If everything is happening in one thread, this is normal. In case of multithreading, when one thread accesses memory, the GIL blocks all other threads. This is a problem for multi-threaded Python programs. It is not a problem for multi-processing Python since each process has its own memory.
How can this issue be resolved? Solutions are to use multiprocessing, use extensions written in C, or use other Python implementations like IronPython, or Cython.
Python Coding
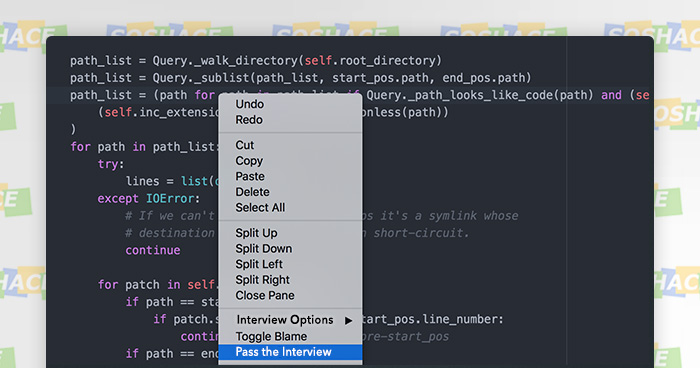
This option is still in beta for now
After testing our candidate’s Python theory and fundamentals knowledge, we offer our interviewees some coding challenges. Whether a whiteboard or a laptop, code is the soul of the programming language — so we try and gauge how effective the job candidate can code.
10. How can we fetch every 5th list element — in different ways?
One option would be this:
1 | [x for i, x in enumerate(thelist) if i%5 == 0] |
Another approach:
1 2 3 | for i, x in enumerate(thelist): if i % 5: continue yield x |
And another:
1 2 3 4 5 | a = 0 for x in thelist: if a%5: continue yield x a += 1 |
11. How can delegation be used? Provide an example
In Python, delegation is a design pattern that allows the developer to change the behavior of a single method inside a given class. To do this, we initialize a new class that creates a new implementation of our method of interest, delegating the rest of the methods to the previous class.
1 2 3 4 5 6 7 | class upcase: def __init__(self, out): self._out = out def write(self, s): self._outfile.write(s.upper()) def __getattr__(self, name): return getattr(self._out, name) |
In this example, the upcase
class changes the file’s contents from lower to upper case. Delegation is done via the self.__outfile
object.
12. How can try… except… can be used efficiently?
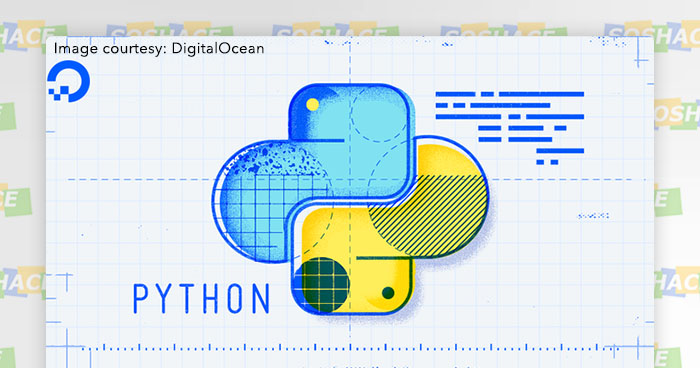
Connecting the code, connecting the world
In some cases, the try… except… combo can replace if… else…; this can be attributed to if statements executing their checks every time they are called, while “ry statements do not utilize checking at all. As an example, we can use a large list of strings containing integers — say, 10,000 strings. We suspect that our list also contains strings with letters and we need to sort them out (or rather ask the user to fix these inputs). Using the if — else block, the “if” check is performed for the whole amount of strings in the list — in our case, this can cause significant load. To skip constant checks, we can utilize try — except and guide the user through our program without stopping it:
1 2 3 4 5 6 7 8 9 10 | list_of_strings = ['25', '26', 'a', '27'] list_of_numbers = [] for num in list_of_numbers: try: list_of_numbers.append(int(num)) except: print('Found incorrect entries (entries containing letters)') # and/or # continue |
The caveat of this method that we cannot learn what type of errors we may be running. Additionally, using the finally
statement, we can make sure that certain resources are released — a good example would be database resources.
13. How can we read/write binary data using Python?
The struct
module can be used for this task. The resulting program will be doing back and forth conversions between binary data and python objects:
1 2 3 4 | import struct f = open(file-name, "rb") s = f.read(8) x, y, z = struct.unpack(">hhl", s) |
14. What will be the output of the following code?
1 2 3 | x = [[]]*3 x[2].append(1) print(x) |
Output:
1 | [[1], [1], [1]] |
15. Write a Python program to fetch the first 7 Fibonacci numbers
Note: the Fibonacci Sequence is the series of numbers: 0, 1, 1, 2, 3, 5, 8, 13, 21, 34, . . . Each subsequent number is the sum of the previous two.
1 2 3 4 | a, b = 0, 1 for i in range(0, 7): print(a) a, b = b, a + b |
16. Given a list of tuples, remove all tuples from the list if any is greater than n. Use filter and lambda
1 2 3 4 | n = 100 init_tuples = [('b', 100), ('c', 200), ('c', 45), ('d', 876), ('e', 75)] result = list(filter(lambda x: x[1] <= n, init_tuples)) print (str(result)) |
Output:
1 | [('b', 100), ('c', 45), ('e', 75)] |
17. What will be the output of the following code?
1 2 3 | a = 1 b = 1 print(a is b) |
Output:
1 | True |
18. Create a prime number list from (1,100)
1 2 3 4 5 6 7 8 9 10 11 12 | primes = [] for possiblePrime in range(2, 100): isPrime = True for num in range(2, possiblePrime): if possiblePrime % num == 0: isPrime = False if isPrime: primes.append(possiblePrime) print(primes) |
Conclusion
To attract the best talent, your company should utilize the best hiring strategy. Hopefully, these top 18 Python interview questions will improve the way you hire Python developers. Whenever you need more insights and guides to maximize your human resources, you know where to find them! (hint: blog.soshace.com 🙂 )
2 comments
Great post i must say and thanks for the information. Education is definitely a sticky subject. However, is still among the leading topics of our time. I appreciate your post and look forward to more.
Really impressed! Everything is very open and very clear clarification of issues. It contains truly facts. Your website is very valuable. Thanks for sharing.